Use NestJs exceptions layer to create a filter that handles all exceptions that are not handled throughout the application.
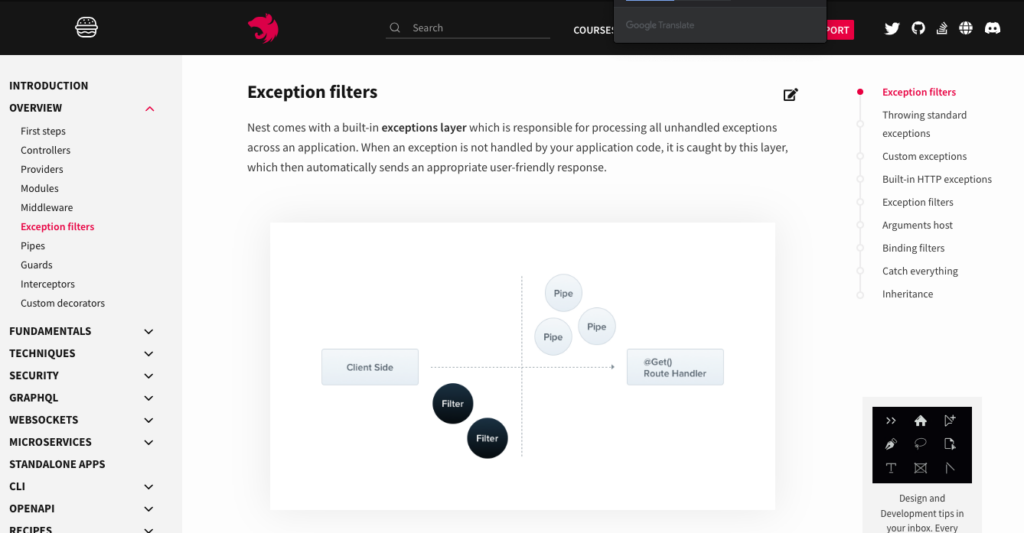
The result should be empty and the data returned.
{
"status": "false",
"errorCode": "E001",
"result": []
}
Define error codes
Define constants.ts.
Add the required error codes.
export default class ErrorCode {
/** 内部サーバーエラー */
static readonly E001 = 'E001';
/** 認証エラー */
static readonly E002 = 'E002';
}
Implement exception filters
The exception filter must implement the ExceptionFilter interface, and the @Catch(HttpException) decorator will bind the necessary data to the exception filter.
If HttpStatus is 500 or higher, set E001 in constants.ts as internal server error, otherwise set thrown exception.message.
import {
ArgumentsHost,
Catch,
ExceptionFilter,
HttpException,
HttpStatus,
} from '@nestjs/common';
import { Response } from 'express';
const responseInterface = {
status: false,
errorCode: '',
result: [],
};
@Catch()
export class HttpExceptionFilter implements ExceptionFilter {
catch(exception: HttpException, host: ArgumentsHost) {
const ctx = host.switchToHttp();
const response = ctx.getResponse<Response>();
const statusCode =
exception instanceof HttpException
? exception.getStatus()
: HttpStatus.INTERNAL_SERVER_ERROR;
responseInterface.status = false;
responseInterface.errorCode = this.setErrorCode(
statusCode,
exception.message,
);
response.status(statusCode).json(responseInterface);
}
private setErrorCode(statusCode: number, message): string {
if (statusCode >= 500) {
return 'E001';
}
return message;
}
}
If you want to return E002 as an authentication error, set the error code to UnauthorisedException and throw it.
throw new UnauthorizedException(ErrorCode.E002);
Commonisation of Responses
For a common Response, see this article.
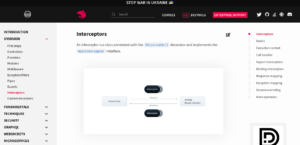